Alright, let’s talk about this little ‘4.1 countdown’ thing I put together today. It wasn’t anything too crazy, just wanted to get a simple timer ticking down on a page.
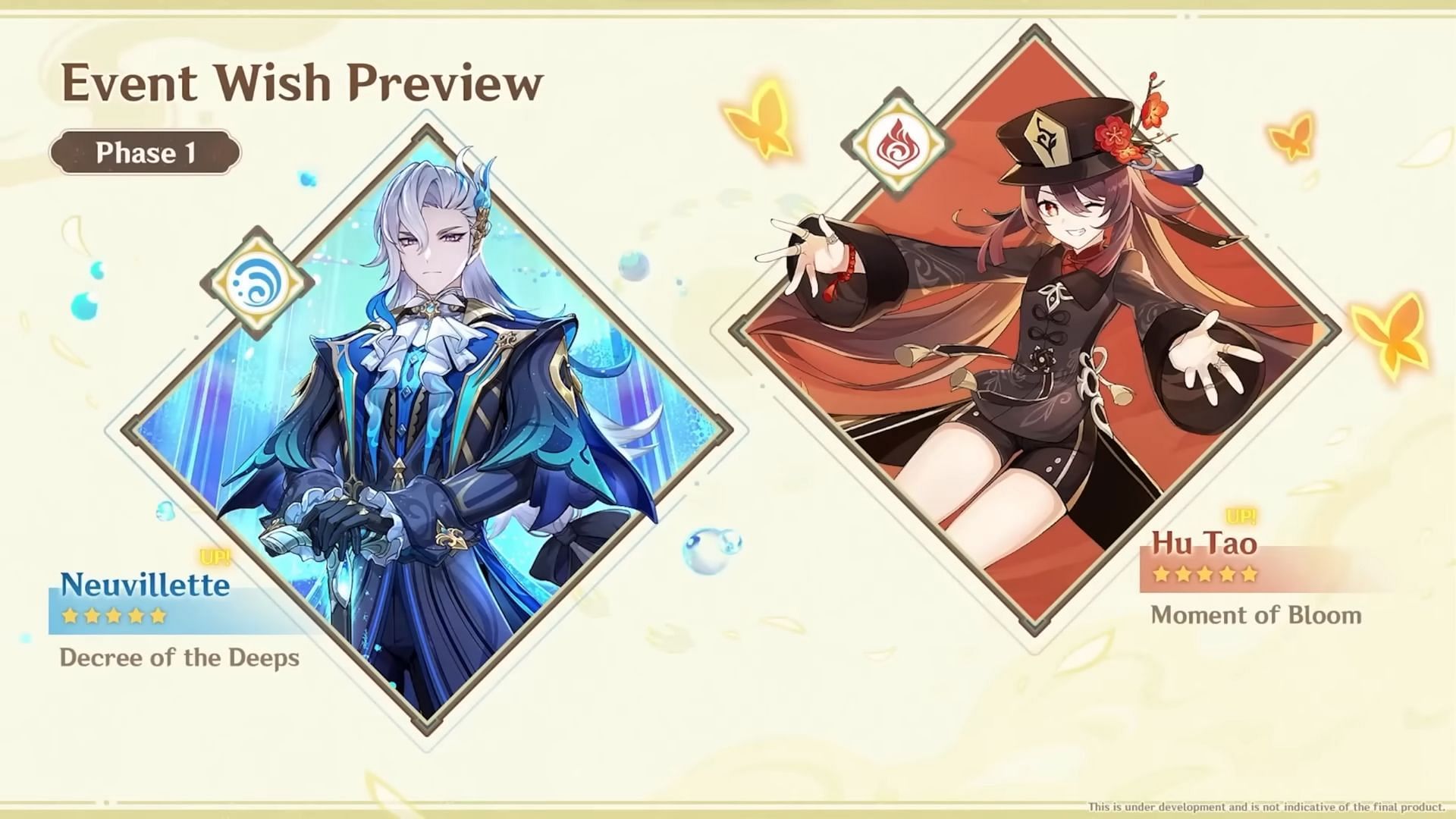
So, first off, I just needed a place to show the numbers, right? I opened up my editor and slapped down some basic HTML. Didn’t need anything fancy, just a plain old paragraph tag, maybe gave it an ID like ‘timer-display’ or something simple so I could grab it later with script.
Getting the Time Stuff Ready
Next up was the brain part – the Javascript. I needed two main things: the date we’re counting down to, and the current date and time. For the target date, I just set it directly in the script. Let’s say it was for April 1st, just picked a date.
Then, inside a function, I got the current time. You know, `new Date()` gives you that right now.
The main bit was figuring out the difference. Just subtract the current time from the target time. That gives you a big number, usually in milliseconds. Not very useful like that.
Breaking Down the Time
So, I had to break down those milliseconds. You know the drill:
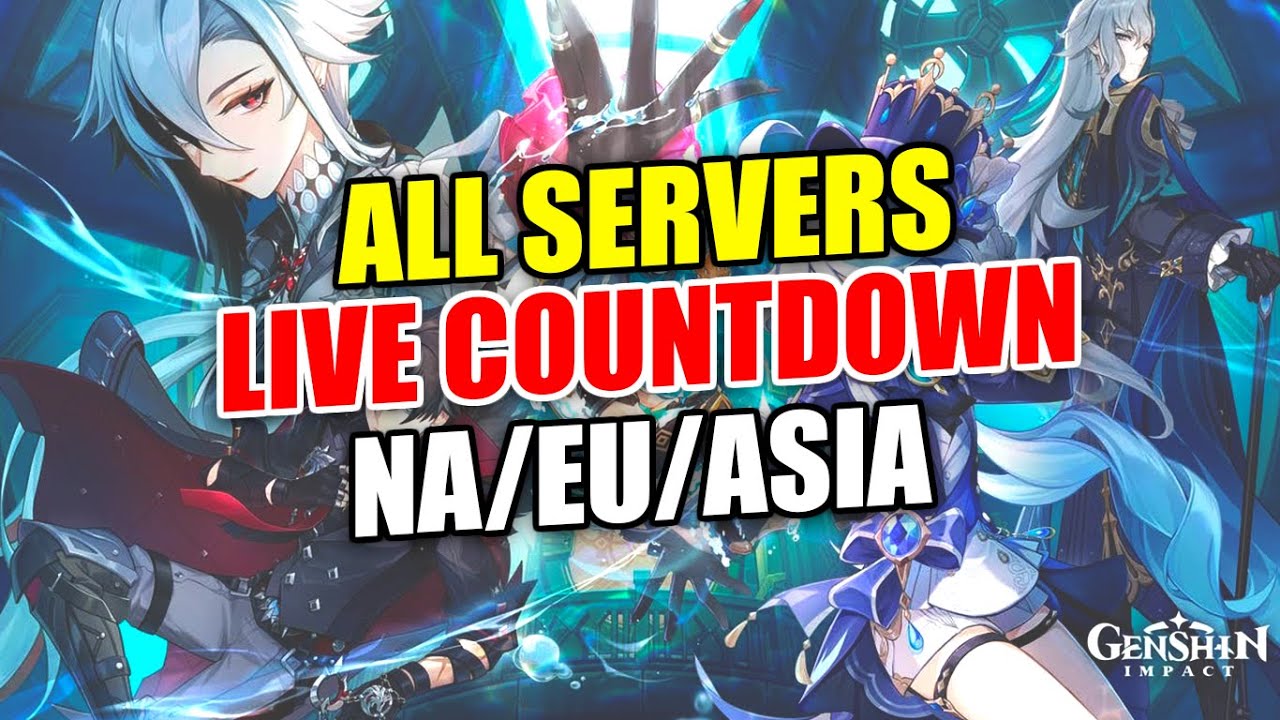
- Divide by 1000 to get seconds.
- Divide seconds by 60 to get minutes.
- Divide minutes by 60 to get hours.
- Divide hours by 24 to get days.
Had to use the modulo operator (%) quite a bit here to get the remainders, like the leftover seconds after calculating minutes, and leftover minutes after calculating hours. A bit fiddly, but standard stuff.
Making it Update Live
A static number isn’t a countdown, right? It needs to tick. So I used that Javascript thing that runs code over and over again, set it to run every second (1000 milliseconds). Inside that repeating function, I did all the time difference calculations I just mentioned.
Then, I took the calculated days, hours, minutes, and seconds, made them into a nice string, and stuffed it back into that paragraph tag I made earlier using its ID. `*(‘timer-display’).innerText = …` or something like that.
Finishing Touches
Looked okay, but single-digit seconds or minutes looked a bit weird, like ‘ H:M:S’. So I added a quick check. If the number (seconds, minutes, etc.) was less than 10, I stuck a ‘0’ in front of it. Makes it look cleaner, like ‘0H:0M:0S’.
Also put in a check for when the countdown finishes. If the time difference calculation resulted in zero or less, I just stopped the repeating timer thing and maybe changed the display message to “Time’s up!” or whatever.
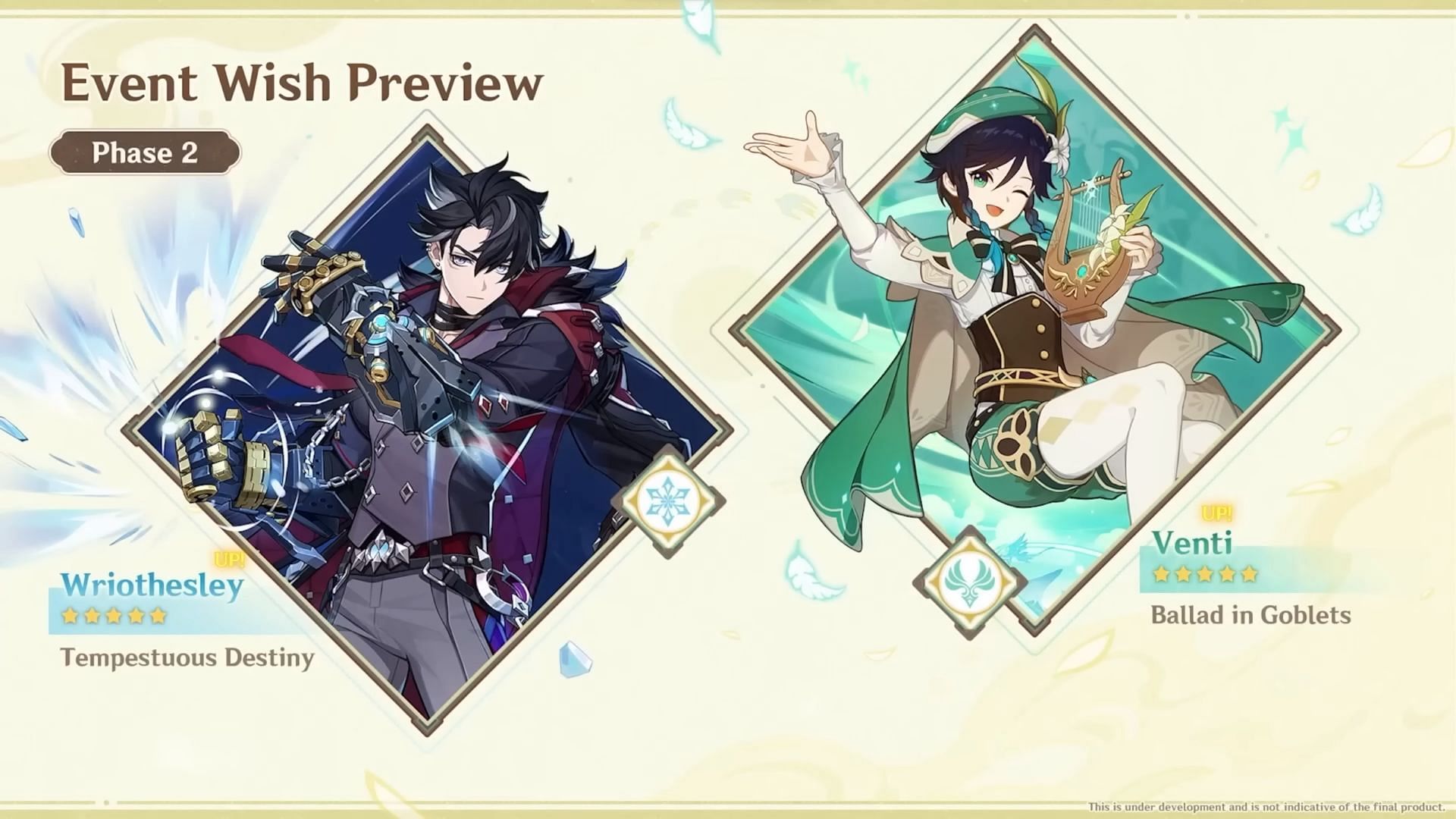
And yeah, that was pretty much it. Got it running. Just a simple countdown, nothing world-changing, but it does the job. Felt good to just build something practical, step-by-step.