Okay, so let me tell you about this “goff super bowl” thing I messed around with. It was a fun little project, nothing too serious, just something to kill some time and learn a bit.
First off, the idea: I wanted to simulate a Super Bowl game using Go, hence “goff” (Go + football, get it? corny, I know). I wasn’t aiming for a super realistic simulation, just something that could randomly generate scores, track possessions, and declare a winner.
Getting Started: I started by setting up my Go environment. I made sure I had Go installed and configured correctly. Then, I created a new directory for the project and initialized a new Go module using go mod init
.
Core Structures: Next, I defined the basic data structures I’d need. I created structs for:
Team
: Had fields like name, points, possession (boolean), and maybe a simple offensive rating.Game
: Held the two teams, the current quarter, and potentially some game log.
Game Logic: This was the meat of the project. I started with functions for:
simulatePlay()
: This was the most basic function. It would randomly generate a result (touchdown, field goal, turnover, etc.) based on the teams’ ratings. It was pretty simple, just a bunch of calls with different probabilities.advanceQuarter()
: Incremented the quarter and printed a little message.switchPossession()
: Switched thepossession
flag between the two teams.
The Main Loop: I then created a main()
function that:
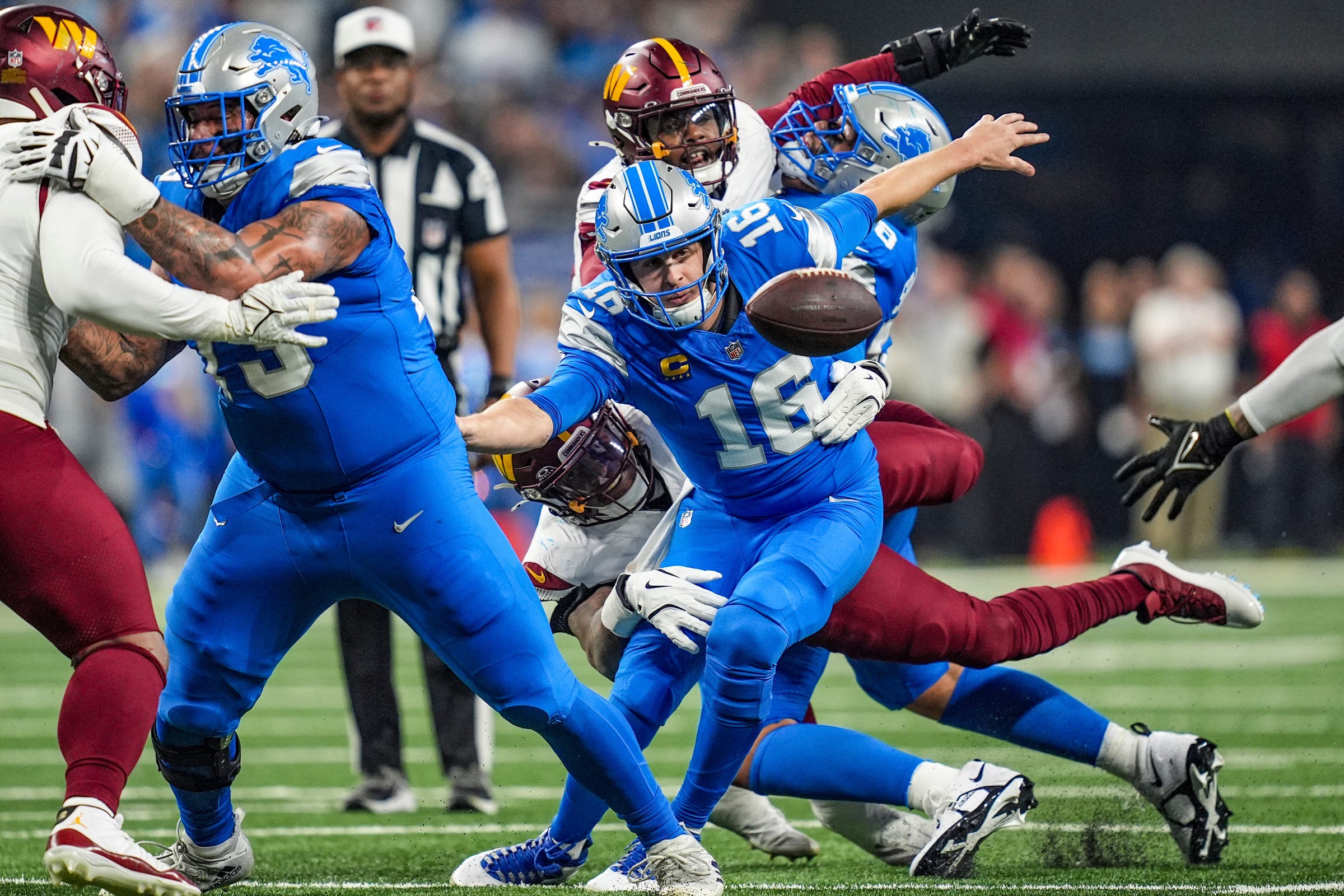
- Initialized the two teams (I just hardcoded some names for now, like “The Go Gophers” and “The Rust Raptors”).
- Started a loop that ran for four quarters.
- Inside the loop, I had another loop that simulated plays until the quarter ended (I just used a fixed number of plays per quarter).
- After each play, I updated the score and printed a summary of the play (e.g., “The Go Gophers gained 7 yards!”).
Generating Random Events: For the simulatePlay()
function, I used the math/rand
package to generate random numbers. I seeded the random number generator using *(*().UnixNano())
to make sure the results were different each time I ran the simulation.
Adding some spice : after the code was running, I added more fun and events to make it feel like a real game.
- Add penalties.
- Add fumble events.
- Add incomplete pass.
Declaring the Winner: After the four quarters were over, I compared the scores of the two teams and printed a message declaring the winner.
Challenges: There were a few hiccups along the way. Getting the random number generation to work the way I wanted was a bit tricky. Also, I had to debug some logic errors in my play simulation code.
What I Learned: This project was a good exercise in basic Go programming. I got more comfortable with structs, functions, loops, and random number generation. It also reinforced the importance of testing and debugging your code.
Future Improvements: If I were to continue working on this, I’d like to:
- Make the play simulation more realistic (e.g., by considering the teams’ strengths and weaknesses).
- Add more detailed play-by-play commentary.
- Implement a simple user interface.
The Code: I’m not gonna paste the whole code here, but it was just a few hundred lines of simple Go. Nothing too fancy.
All in all, the “goff super bowl” was a fun little diversion. It wasn’t going to win any awards, but it was a good way to spend a weekend and learn a bit more about Go.