Okay, so today I wanna share how I tackled this problem, felt like I was banging my head against a wall for a while there. The title I’m giving it is “find a way to open the path forward”. Sounds kinda dramatic, but trust me, it felt that way at the time.
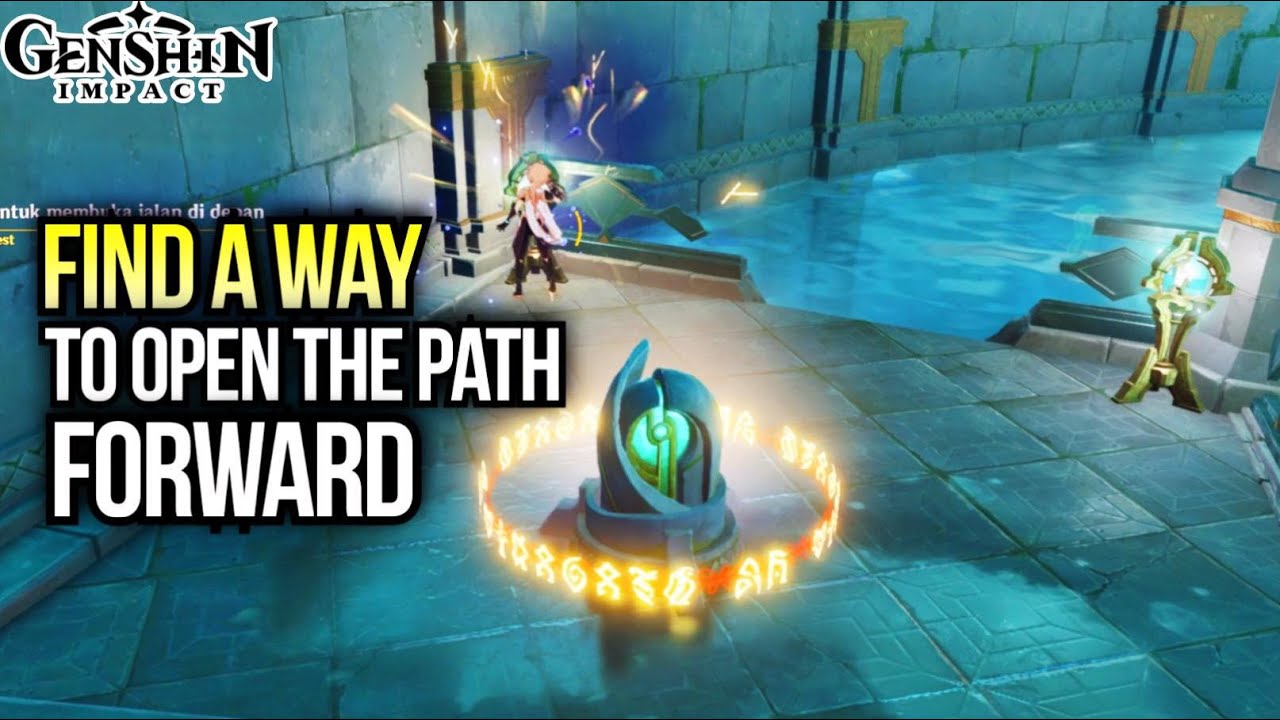
The Initial Problem: I was stuck on this project, right? Basically, I needed to get data from one system and push it into another, but the two systems spoke completely different languages. System A was all about XML, and System B wanted JSON. Plus, the data structures were, like, totally different. Think trying to fit a square peg in a round hole… repeatedly.
First Attempt: Naive Approach So, naturally, I started with the most obvious thing: writing a script to manually parse the XML, transform it, and then build the JSON. I chose Python since I knew my way around it and figured, “How hard could it be?” Famous last words, right?
- I grabbed the XML data using the `requests` library.
- I started parsing it with `*`.
- Then I tried to map the XML elements to the JSON structure.
It quickly turned into a mess of nested loops, conditional statements, and string manipulation. I spent hours debugging, and every time I fixed one thing, three more things broke. It was fragile, slow, and frankly, a nightmare to maintain.
The “Aha!” Moment: Rethinking the Strategy After a couple of days of frustration, I stepped back and thought, “There HAS to be a better way.” I realized I was trying to do everything myself, reinventing the wheel at every turn. That’s when I started looking for tools that could handle the transformation for me.
Enter JOLT: The JSON to JSON Transformation Tool I stumbled upon JOLT (JSON to JSON Transformation) from Braintree. It’s basically a JSON transformation library where you define a “spec” that describes how to transform the input JSON into the desired output JSON. It seemed perfect, but there was a catch: I still had XML.
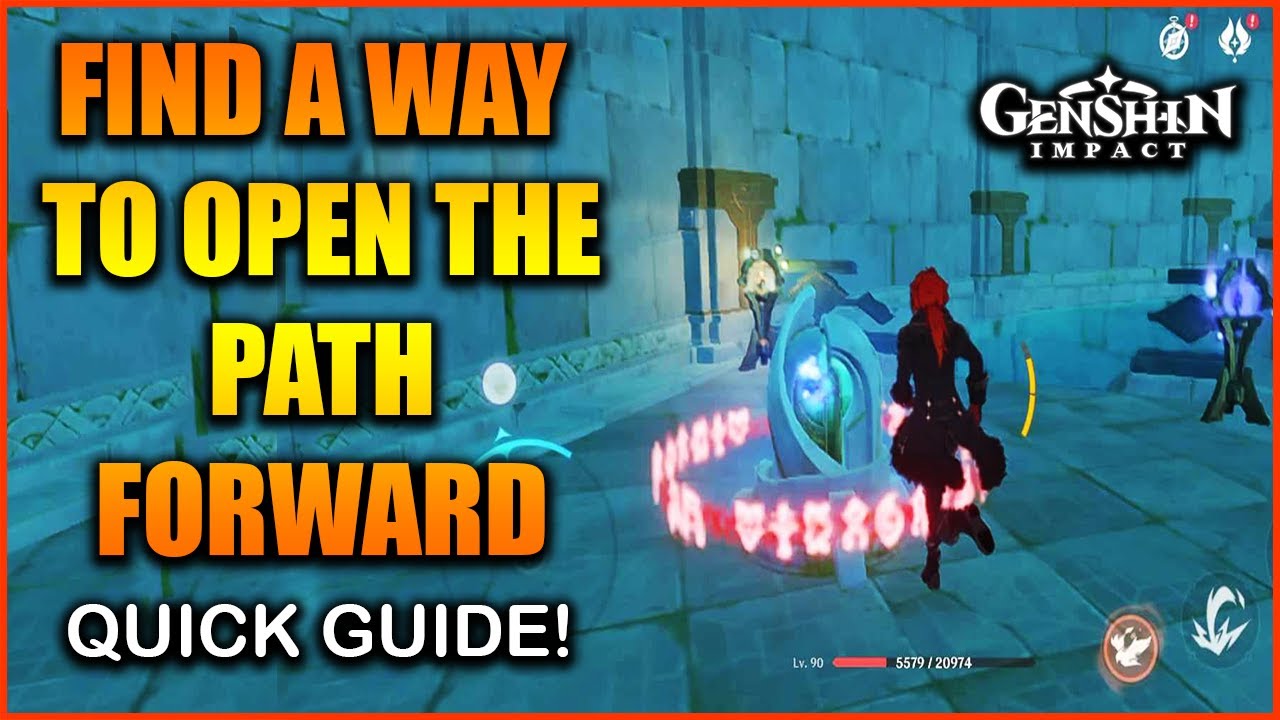
Bridging the Gap: XML to JSON So, the new strategy became: 1. Convert XML to JSON. 2. Use JOLT to transform the JSON. I found a few Python libraries that could handle the XML to JSON conversion. I ended up using `xmltodict` because it seemed relatively straightforward and produced clean JSON.
- I installed `xmltodict`: `pip install xmltodict`
- I loaded the XML data with `requests`.
- I converted it to JSON using `*()`.
JOLT to the Rescue: Defining the Spec Now, the real magic happened. I spent some time crafting the JOLT spec. It took a bit of experimenting to get it right, but once I understood the syntax, it was surprisingly powerful. I could define how to rename fields, flatten nested structures, and even conditionally transform data based on its values.
The Workflow Here’s the final flow I ended up with:
- Fetch the XML data from System A.
- Convert the XML to JSON using `xmltodict`.
- Apply the JOLT spec to transform the JSON into the format expected by System B.
- Send the transformed JSON to System B using the `requests` library.
The Result: Success! It worked! The transformation was now much cleaner, faster, and easier to maintain. The code was more readable, and I could easily modify the JOLT spec to handle changes in the data structures. The entire process was less fragile and far less prone to errors.
Lessons Learned:
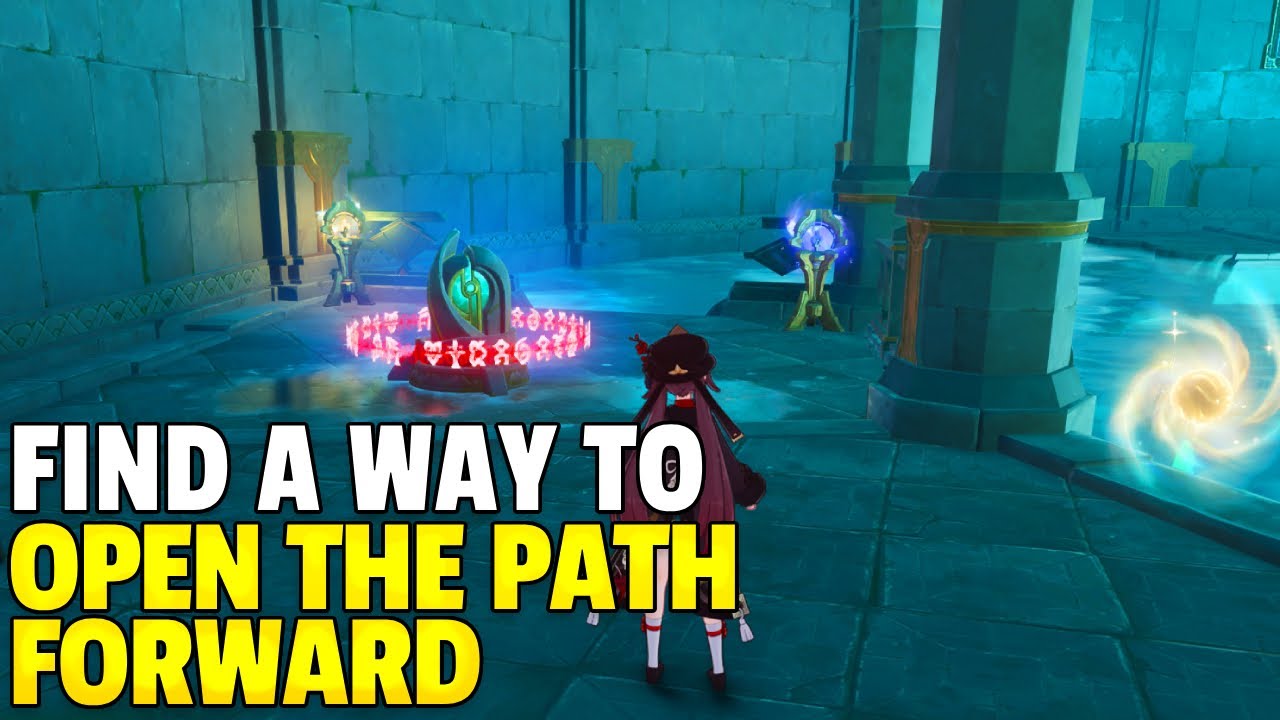
- Don’t be afraid to look for existing tools: I wasted a lot of time trying to reinvent the wheel. Sometimes, the best solution is to leverage existing libraries and frameworks.
- Break down the problem: Instead of trying to solve everything at once, I broke the problem into smaller, more manageable pieces (XML to JSON, then JSON transformation).
- Experiment and iterate: The JOLT spec wasn’t perfect on the first try. I had to experiment and iterate until I got it right.
Final Thoughts: Sometimes, the path forward isn’t immediately obvious. It requires stepping back, rethinking the problem, and being willing to try new approaches. This experience taught me the importance of not being afraid to explore different tools and techniques, and to always be open to learning new things. It was a pain at first, but I’m definitely glad I stuck with it. Now I can open that path forward more easily the next time!