Okay, so I was messing around with some data the other day and decided to tackle something I’ve been putting off for ages: building my own database (DB) for fantasy football rankings. Here’s how I did it, from zero to… well, slightly-better-than-zero.
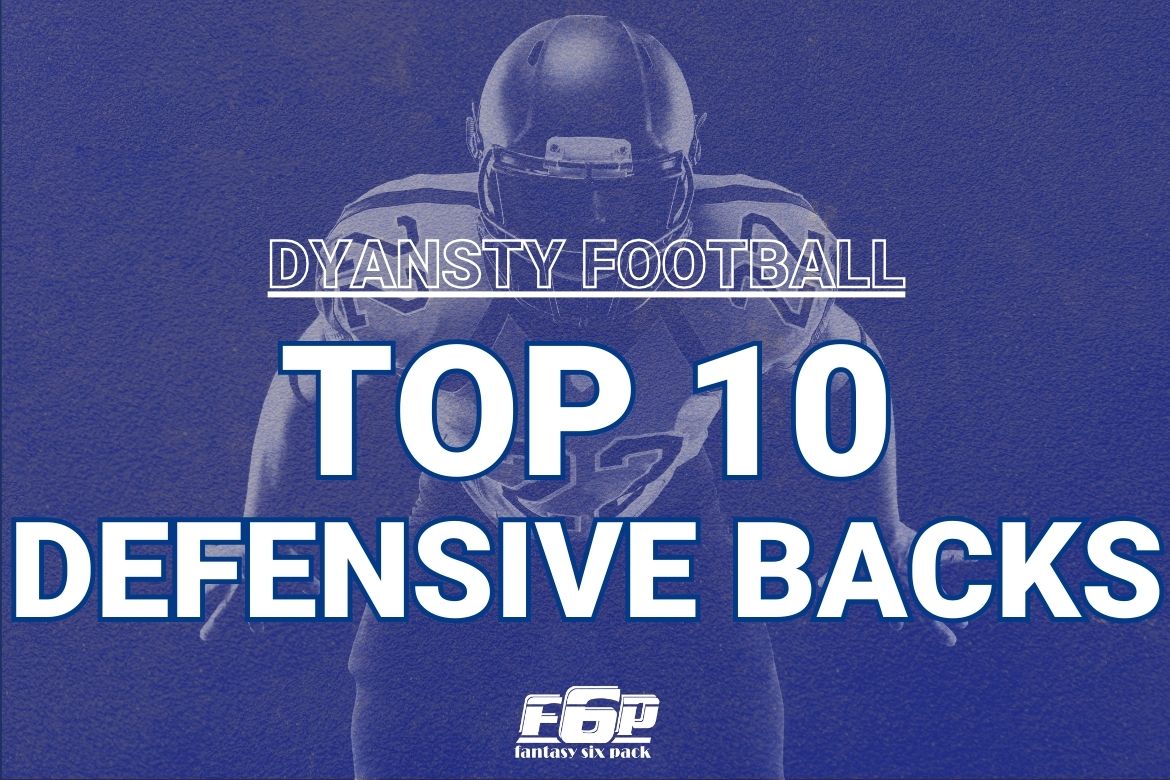
Step 1: Data Gathering – The Scavenger Hunt
First things first, I needed data. Lots of it. I started by hitting up a bunch of sports websites. You know the usual suspects: ESPN, CBS Sports, maybe even some smaller, more niche fantasy football sites. I was looking for anything and everything: player stats from last season, projected stats for the upcoming season, expert rankings, average draft positions (ADP), injury reports – the whole shebang. I literally copied and pasted a lot of data into a text file to start.
Step 2: Cleaning Up the Mess – Excel is Your Friend (Maybe)
Oh man, data cleaning. This part sucks. So much copy-pasting gave me a HUGE messy file. I dumped all that raw, unformatted text into an Excel spreadsheet. Then the real fun began. I had to manually go through each column, separate names, positions, teams etc. I used Excel functions like `LEFT`, `RIGHT`, `MID`, and `FIND` like crazy to chop things up. It was tedious, but necessary. I really needed to get everything into a structured format, for example, player name in one column, position in another, team in another, then stats like rushing yards in different columns, passing yards, etc.
Step 3: Building the Database – SQLite to the Rescue
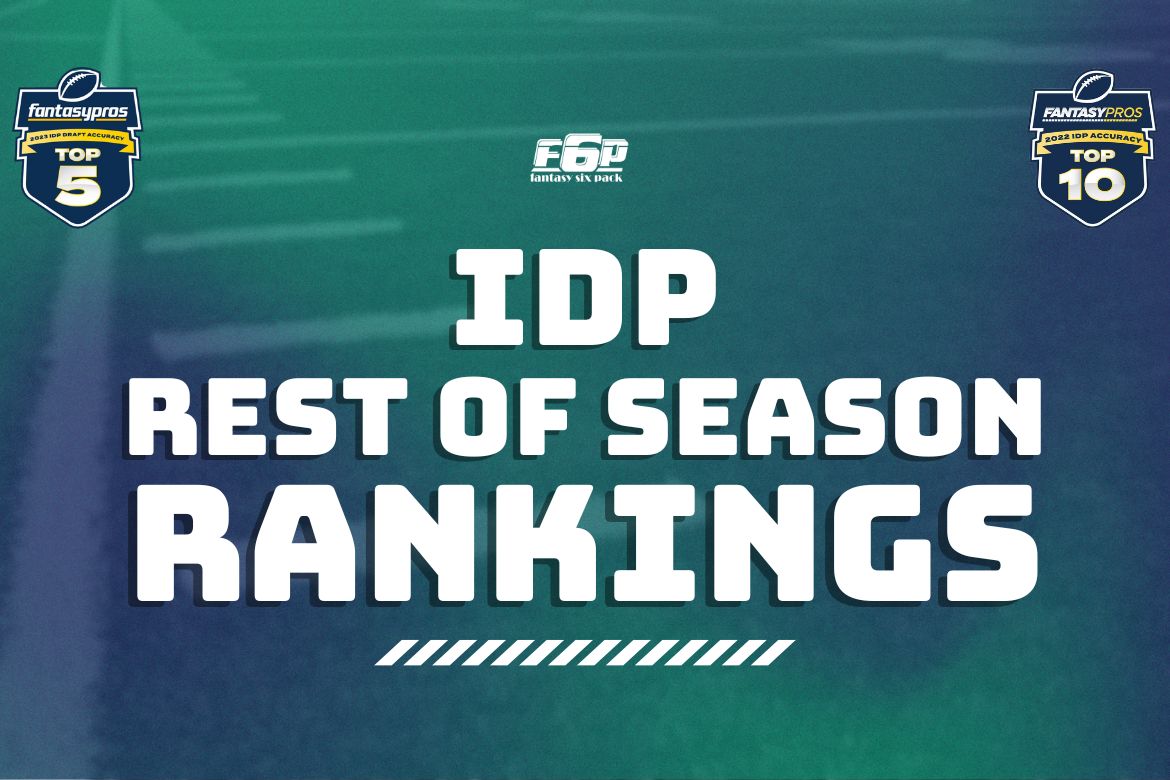
I decided to keep things simple and go with SQLite. It’s lightweight, file-based, and perfect for a personal project like this. I used a Python script with the `sqlite3` library to create a database file and a table called `players`. The table had columns for all the stuff I’d cleaned up in Excel: `player_name`, `position`, `team`, and then a bunch of stat columns like `passing_yards`, `rushing_yards`, `receiving_touchdowns`, etc. Plus a column for my own calculated ranking. Then I used `INSERT` statements to get my data from the Excel sheet and put it into the DB.
Step 4: Ranking Algorithm – My Secret Sauce (Not Really)
Okay, so this is where it gets a little subjective. I created a simple ranking algorithm based on a weighted sum of different stats. For example, I might give passing yards a weight of 0.1, rushing yards a weight of 0.2, and touchdowns a weight of 0.5. Then, for each player, I would multiply their stats by the corresponding weights and sum them up to get their overall score. I stored this score in the `ranking` column in the database. To keep things simple at the start, I hardcoded the weights in the python script, no need to overcomplicate things.
Step 5: Querying and Sorting – Making Sense of it All
Now that the data was in the database and the rankings were calculated, I could start querying it. I wrote some SQL queries to sort the players by their ranking and filter them by position. For example, I could get the top 10 quarterbacks, or the top 20 running backs. This was pretty cool, because now I had a personalized ranking system that I could use to inform my draft picks.
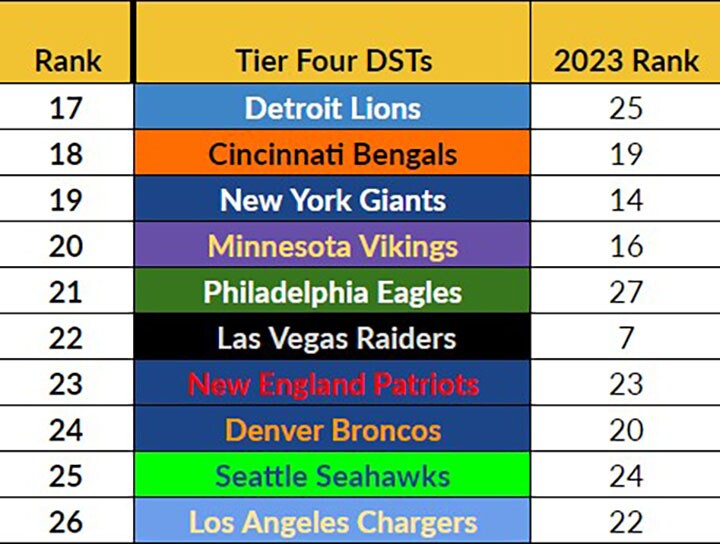
Step 6: Visualizing the Data – Because Numbers are Boring
To make things a bit more visually appealing, I used Python with the `matplotlib` library to create some charts and graphs. I made a bar chart showing the top 10 players overall, and scatter plots showing the relationship between different stats and the ranking. This helped me to get a better sense of which stats were most important for predicting fantasy football success.
Step 7: Iteration and Improvement – The Never-Ending Story
This is an ongoing process. I’m constantly tweaking the ranking algorithm, adding more data, and improving the visualization. I’m also thinking about adding more features, like the ability to compare players head-to-head, or to create custom rankings based on different league settings.
What I Learned
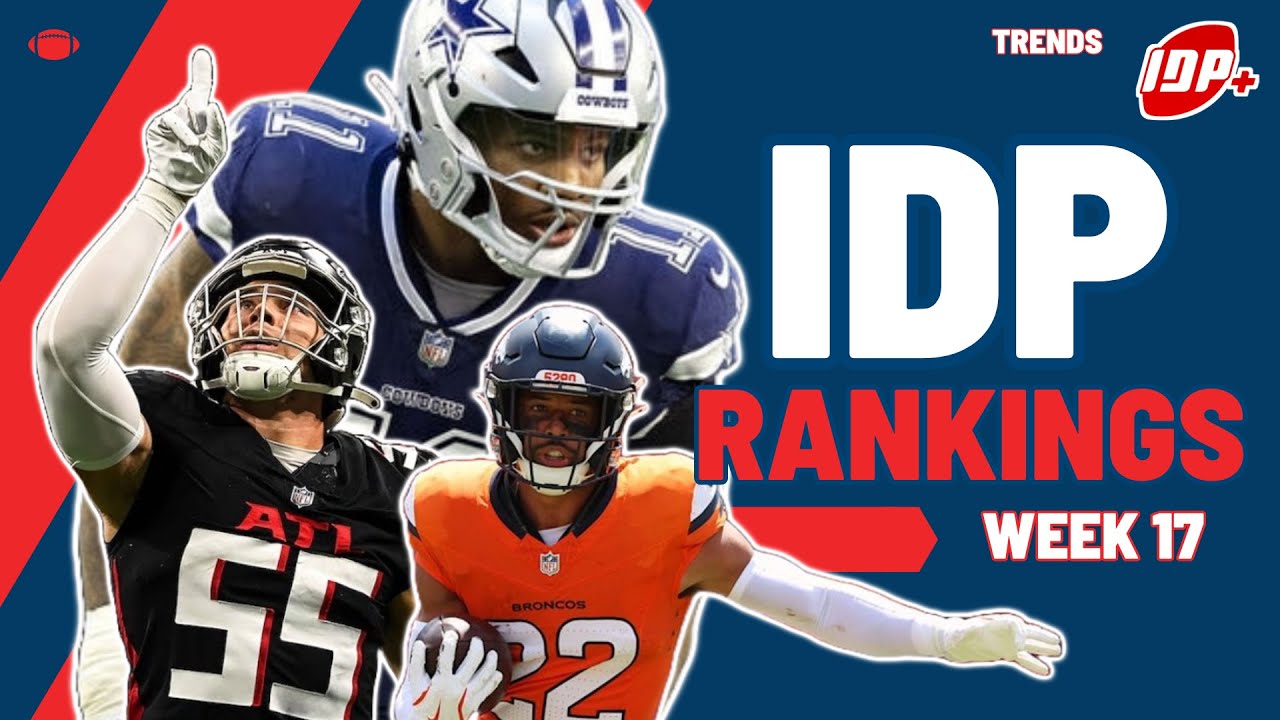
- Data cleaning is a pain, but it’s essential. Garbage in, garbage out.
- SQLite is a great option for small, personal projects. It’s easy to use and doesn’t require a lot of setup.
- A simple ranking algorithm can be surprisingly effective.
- Visualizing data can help you to understand it better.
Next Steps
Gonna keep messing with it, maybe add a web interface using Flask or something. It’s a fun little project!