Alright folks, let me walk you through this little project I tackled recently – I’m calling it “confuses crossword”. Sounds fancy, right? Well, it’s just a crossword puzzle generator that throws in some curveballs.
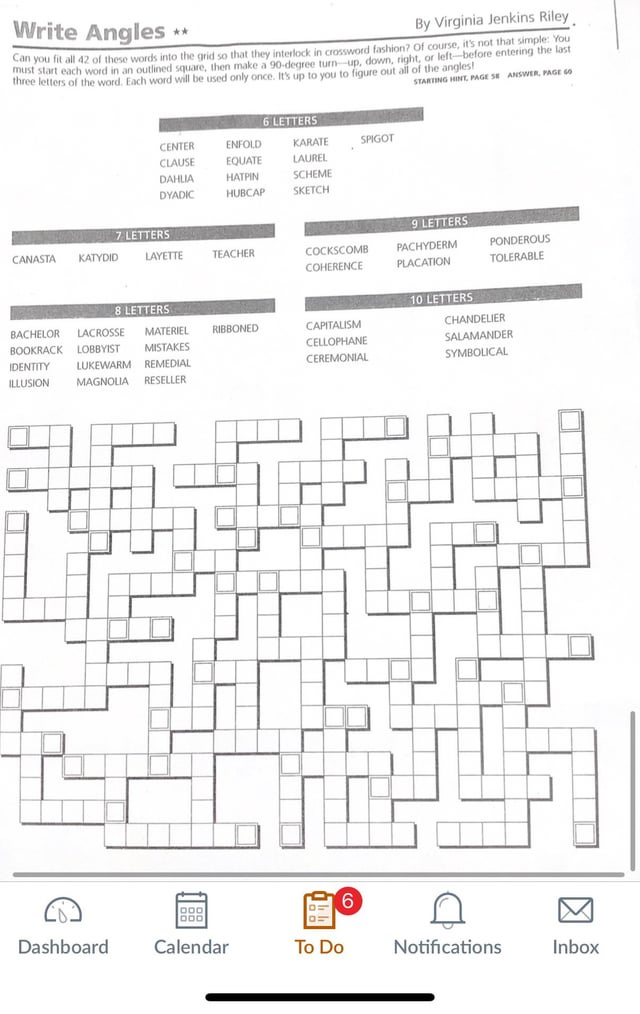
So, I started with the basics. I mean, you gotta crawl before you can run, right? I grabbed a word list – just a plain text file with a bunch of words, one per line. Nothing too crazy. Then, I needed a way to represent the crossword grid. I opted for a simple 2D array, you know, rows and columns. Figured that’d be easy enough to manipulate.
Next up was the fun part: placing the words. This is where things started to get interesting. My initial approach was pretty straightforward. I’d pick a word at random, then try to find a suitable spot on the grid. A “suitable spot” meant either an empty space or a letter that matched the first or last letter of my word. If I found a match, boom, I’d place the word either horizontally or vertically. If not, I’d try a different spot, or even a different word altogether.
But here’s the thing: that approach sucked. It worked for a few words, but then it’d get stuck. The grid would fill up quickly, and I couldn’t find any more valid placements. So, I had to get smarter. I introduced a scoring system. Each potential placement would get a score based on how many letters it shared with existing words, and how much empty space it filled up. I’d then pick the placement with the highest score.
That helped a bit, but it still wasn’t perfect. The algorithm was still getting stuck, especially with longer words. So, I added a backtracking mechanism. If I couldn’t place a word after a certain number of tries, I’d remove the last few words I placed and try again. This gave the algorithm a chance to “undo” bad decisions and explore different possibilities.
Now, for the “confuses” part. I wanted to make the crossword a bit more challenging, a bit more… unexpected. So, I decided to add some tricks. First, I introduced synonyms. Sometimes, the clue would be a synonym of the actual word. For example, the clue might be “big” but the answer would be “large”.
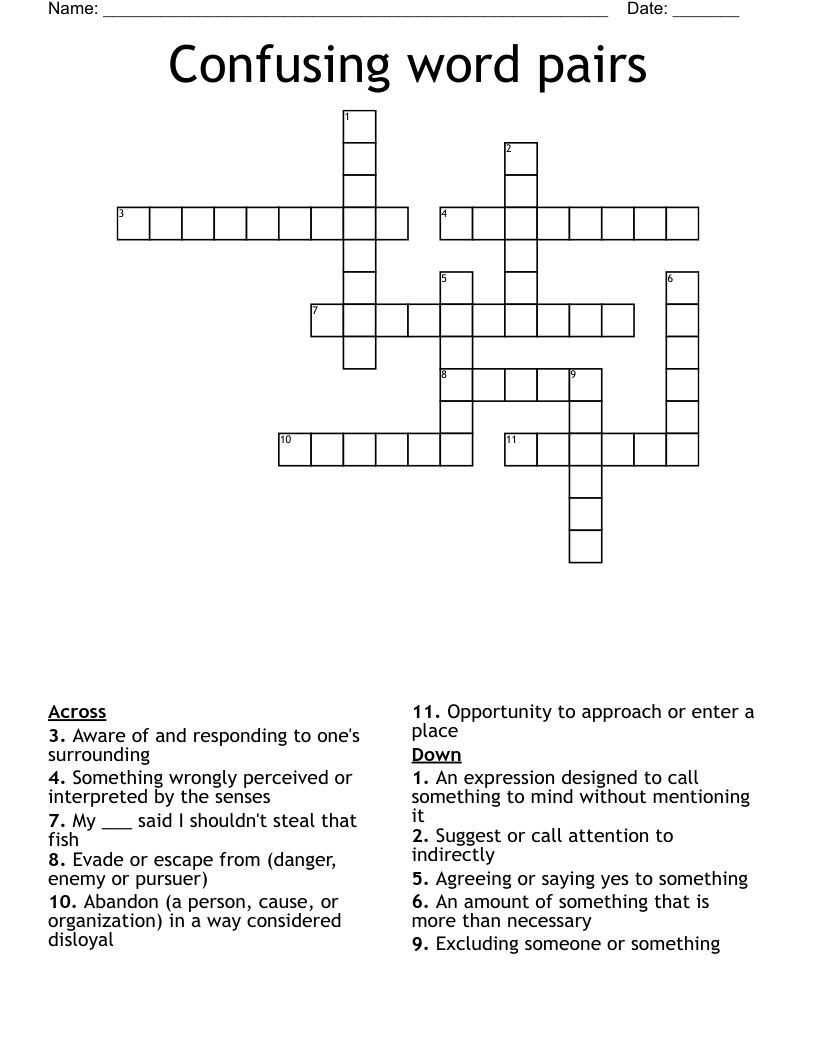
Then, I threw in some red herrings. I’d generate clues that sounded like they were related to a specific word, but actually weren’t. This was tricky to implement, because I had to make sure the red herring clues were plausible, but still misleading.
Finally, I added some fill-in-the-blank clues. These were just sentences with a missing word, and the crossword grid would contain the missing word. This added a different type of challenge, because the user had to understand the sentence in order to figure out the answer.
Once I had all the pieces in place, I built a simple command-line interface to generate and display the crosswords. It’s nothing fancy, but it gets the job done. You give it a word list, and it spits out a crossword puzzle, complete with clues.
Of course, there were plenty of bugs and challenges along the way. I spent a lot of time debugging the word placement algorithm, tweaking the scoring system, and coming up with clever clues. But in the end, it was all worth it. I’ve got a crossword generator that can create some pretty challenging and confusing puzzles. And that, my friends, is a win in my book.
Here’s a quick rundown of the key steps:
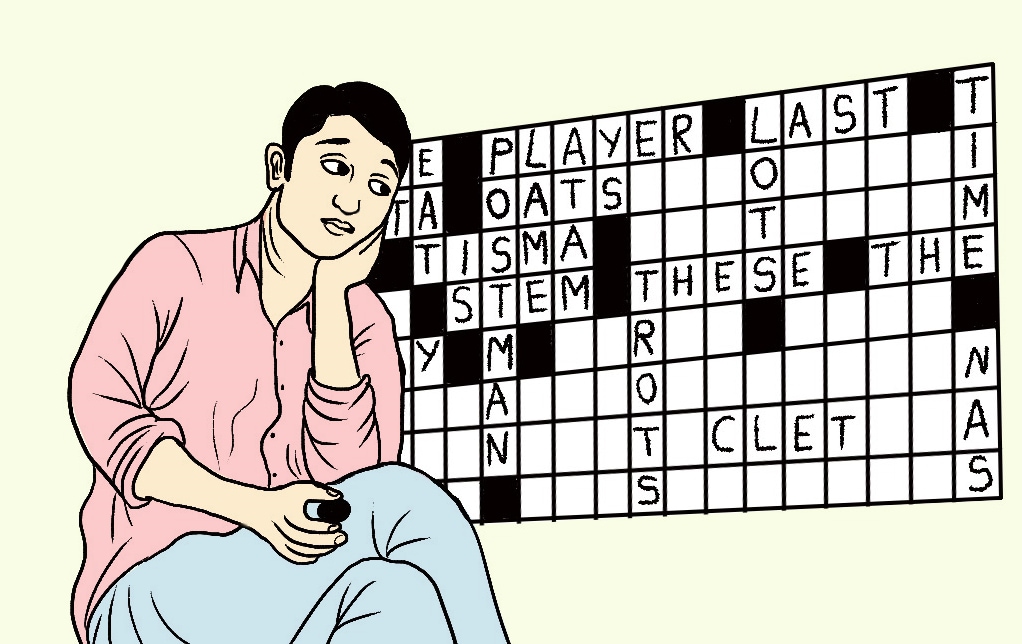
- Loaded a word list.
- Created a 2D array to represent the grid.
- Implemented a word placement algorithm with scoring and backtracking.
- Added synonym clues, red herrings, and fill-in-the-blank clues.
- Built a command-line interface to generate and display the crosswords.
It wasn’t always smooth sailing, but hey, that’s what makes it fun, right?