Alright, let’s dive into this “baltimore orioles vs yankees match player stats” thing. I was messing around with some sports data the other day, and thought it’d be cool to try and pull some player stats for a specific game. Here’s how I tackled it.

First Things First: Data Source
Okay, so the biggest hurdle is always where to get the data, right? I started by just googling around for “MLB stats API” and stuff like that. Found a few options, some free, some paid. I was just experimenting, so I went with a free one that seemed reasonably complete. Can’t remember the exact name right now, but there are tons out there – just gotta find one that works for you.
Getting My Hands Dirty with the API
Once I had the API, I needed to figure out how to actually use it. Most APIs have documentation, which, let’s be honest, can be a pain to read. But gotta do it! I skimmed through it to find the endpoints for getting game data and player stats.
- I figured out how to filter by date to find the specific Orioles vs. Yankees game I wanted.
- Then, I looked for how to get the player stats for that game. Usually, it involves sending a request with the game ID.
I ended up using Python with the `requests` library – pretty standard stuff. I wrote a quick script to:
- Send a request to the API to get the game ID for the Orioles vs. Yankees match on the date I chose.
- Use the game ID to send another request to get all the player stats for that game.
Parsing the JSON
The API spits out data in JSON format, which is basically a fancy way of saying it’s a bunch of nested dictionaries and lists. So, I needed to parse that stuff. Python’s `json` library makes this super easy.
python
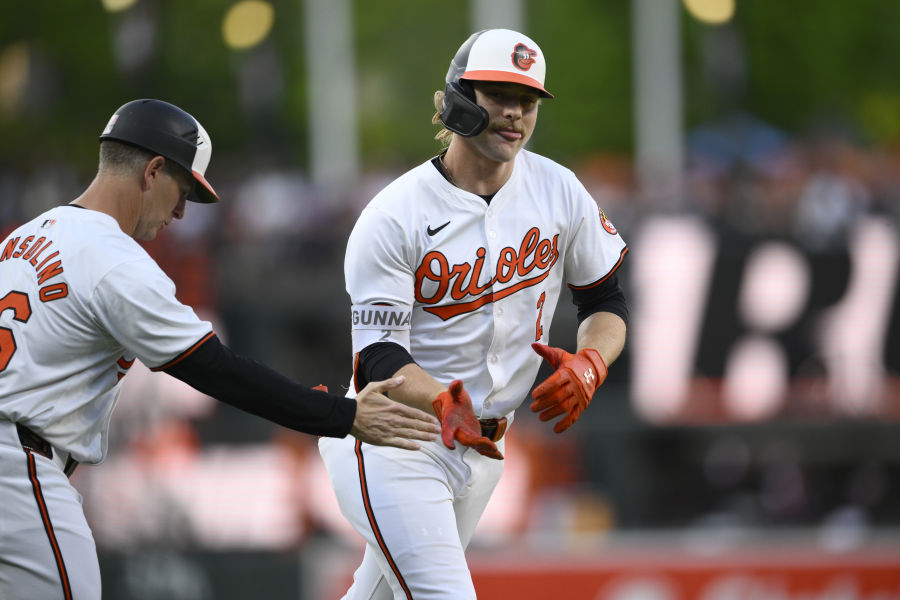
import requests
import json
# Assuming you have the API endpoint and game ID
api_url = “YOUR_API_ENDPOINT” # Replace with the actual API endpoint
game_id = “YOUR_GAME_ID” # Replace with the actual game ID
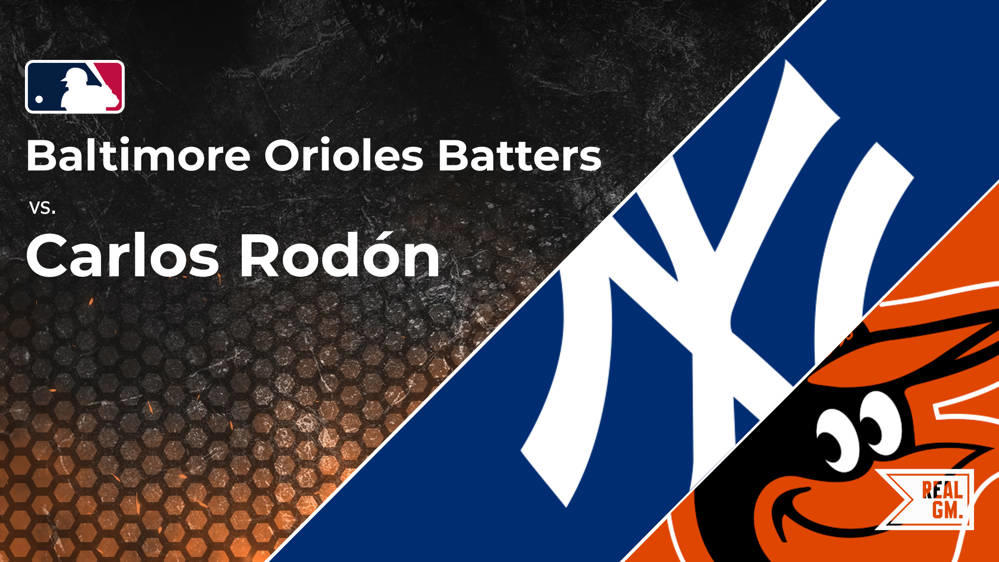
# Construct the URL to get player stats for the game
stats_url = f”{api_url}/game/{game_id}/stats”
# Send the request
response = *(stats_url)
# Check if the request was successful
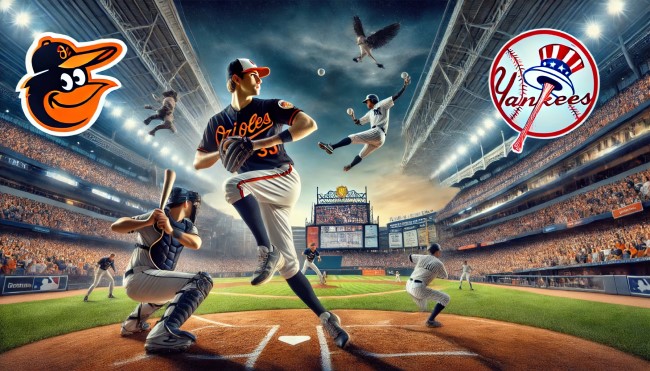
if *_code == 200:
# Parse the JSON data
data = *(*)
# Now you can access the player stats from the ‘data’ dictionary
# For example, to print the names of all players:
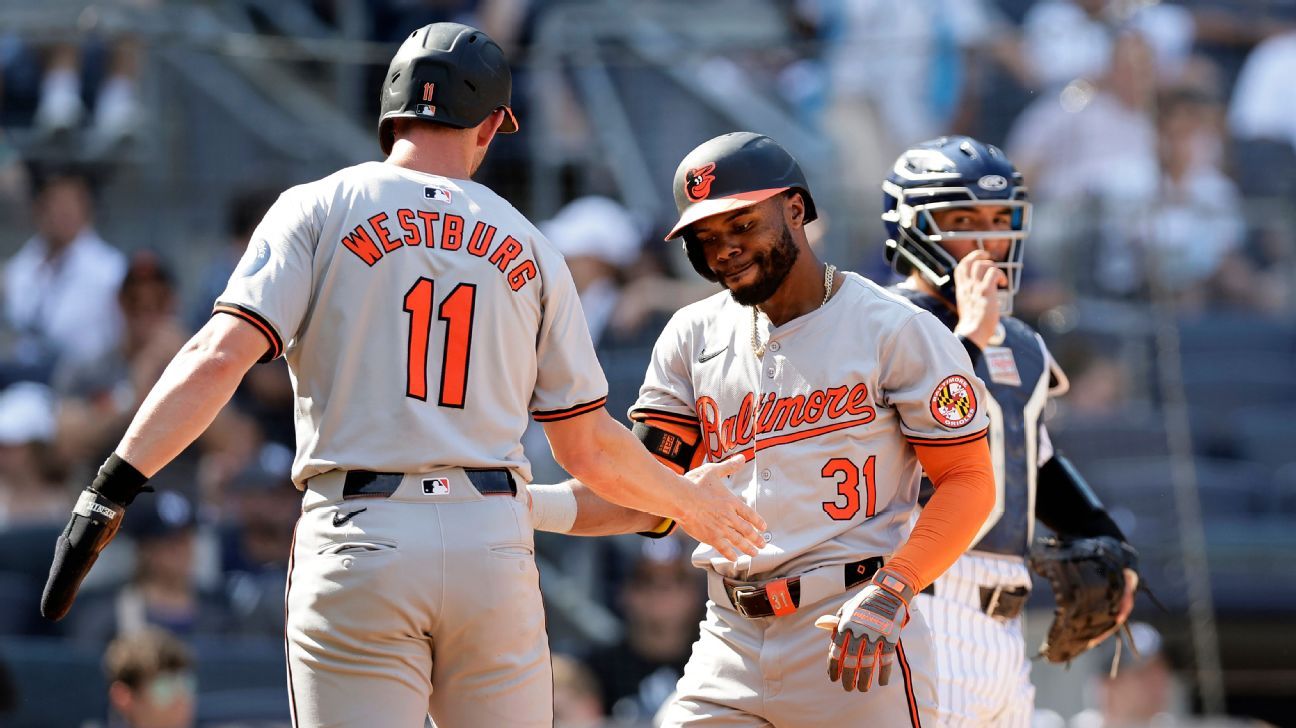
for player in data[‘players’]: # Assuming the data structure has a ‘players’ key
print(player[‘name’]) # Assuming each player has a ‘name’ key
else:
print(f”Error: {*_code}”)
I had to dig around in the JSON to figure out where the player names, batting averages, RBIs, etc. were located. It’s usually a nested structure, so you gotta poke around with `data[‘some_key’][‘another_key’]` until you find what you’re looking for.
Cleaning and Displaying the Data
The raw data from the API is often messy. For example, some values might be missing, or in a weird format. I did a little bit of cleaning to make the data more presentable. This involved things like:
- Checking for `None` values and replacing them with `0` or `”N/A”`.
- Formatting numbers to a specific number of decimal places.
Finally, I just printed the data to the console in a readable format. I could have also saved it to a CSV file or put it in a database, but I just wanted to see the stats, so printing was good enough.
What I Learned
This whole thing was a good reminder that working with APIs can be messy. You gotta deal with authentication, rate limiting, weird data formats, and all sorts of other headaches. But it’s also super powerful, because you can get access to tons of data that you wouldn’t otherwise have.
If I were to do this again, I’d probably spend more time looking for a really good API with clean documentation. That would save a lot of time in the long run. Also, I’d probably use a library like `pandas` to make the data cleaning and manipulation easier. But for a quick little project, it was fun!
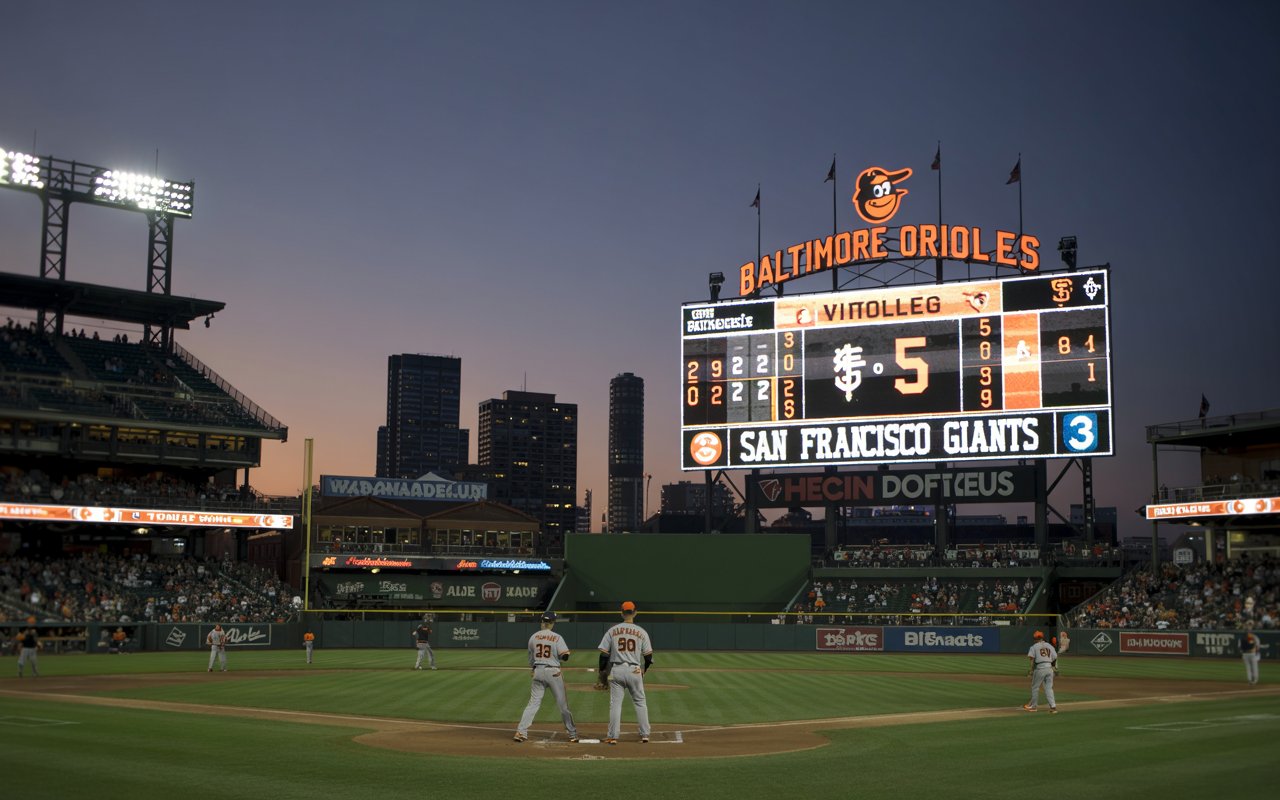
That’s pretty much it. Hope that was helpful! Just a little peek into how I mess around with data. Maybe it’ll inspire you to try something similar!