Alright folks, lemme tell you about this thing I was messing around with called ‘actor mcgregor’. Sounds fancy, right? It ain’t. It’s just something I cooked up to try and get a handle on, well, actors. Not the Hollywood kind, the code kind.
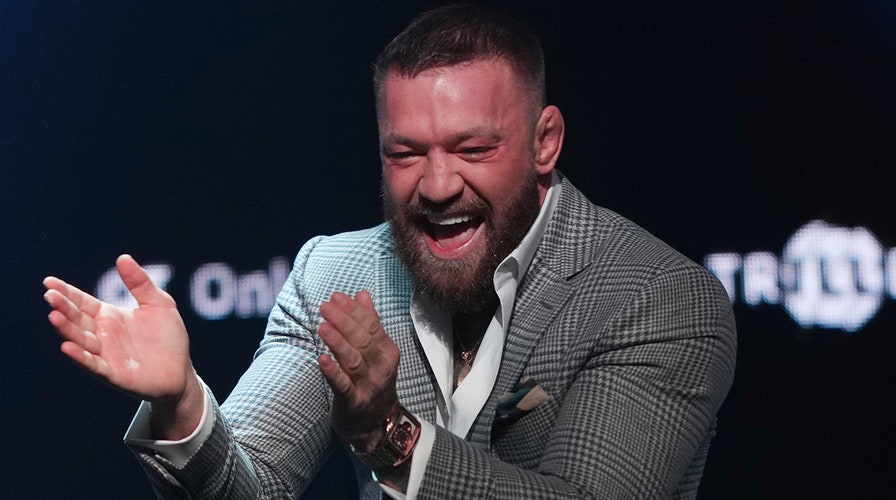
So, I kicked things off by sketching out what I actually wanted this ‘actor’ thing to do. I started simple. Real simple. I’m talking basic ‘receive a message’ and ‘do something with it’ level simple. I figured if I couldn’t nail that, there was no point in even trying to make it more complicated.
First, I needed a way to, you know, create an actor. I wrote up a little function that basically sets up the actor’s initial state and gets it ready to receive messages. Think of it like setting the stage. Nothing on it yet, just… there.
Then came the interesting bit: the message queue. This is where all the action happens. I decided to use a simple list (yeah, yeah, I know, not the most efficient thing in the world, but hey, gotta start somewhere!). When a message comes in, it gets stuck on the end of this list.
Now, how does the actor actually process those messages? That’s where the main ‘loop’ comes in. This loop basically sits there, constantly checking if there are any messages in the queue. If there are, it grabs the first one, figures out what it’s supposed to do with it (based on the message type), and then… does it!
The Core Loop:
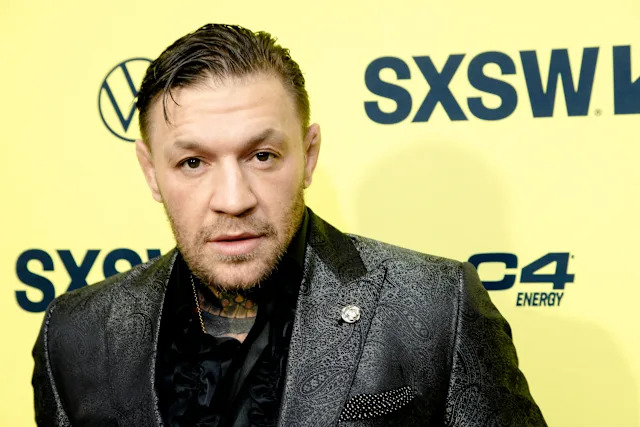
- Check if the message queue is empty.
- If not empty:
- Grab the first message.
- Figure out what function to call based on the message type.
- Call that function with the message data.
I spent a good chunk of time just debugging this loop. Turns out, getting the message handling right is trickier than it sounds. Little things like making sure you’re passing the right data to the right function, handling errors gracefully… yeah, that stuff adds up.
After I got the basic message passing working, I started adding in some ‘actions’. These are just functions that the actor can perform when it receives a specific type of message. For example, I had one that just printed the message to the console. Super useful for debugging!
But the real test came when I tried to get two actors talking to each other. This is where things got interesting. I had to figure out how to send a message from one actor to another, without them blocking each other. Ended up using a simple message-passing system where one actor adds a message to the other actor’s queue.
Actors communicating:
- Actor A wants to send a message to Actor B.
- Actor A creates a message object.
- Actor A finds Actor B’s message queue.
- Actor A appends the message to Actor B’s queue.
- Actor B (eventually) processes the message.
It’s a bit clunky, I admit. But it worked! I had two actors pinging messages back and forth to each other. Felt like a real win.
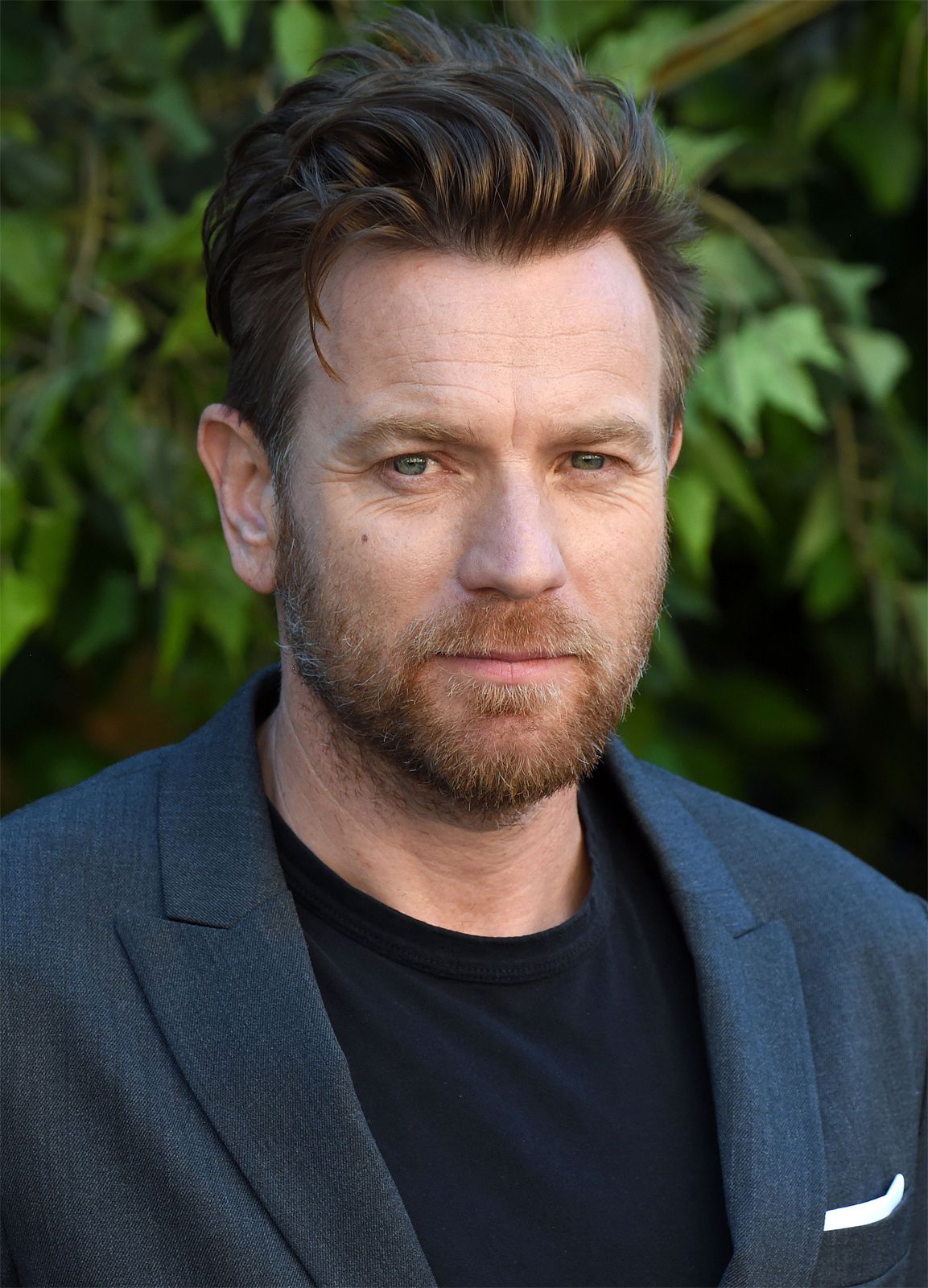
Now, I’m not gonna lie, this ‘actor mcgregor’ thing is still pretty rough around the edges. It’s definitely not ready for production. But it was a fun exercise in getting to grips with the basic concepts of actor-based concurrency. I learned a lot about message queues, event loops, and the joys (and pains) of trying to get multiple things to happen at the same time.
So yeah, that’s ‘actor mcgregor’ in a nutshell. A bit of a mess, but a mess that taught me something. And that’s what it’s all about, right?