Okay, so, yesterday I was messing around with some sports data, trying to get a handle on player stats from that Colorado Rockies versus Padres game. You know, just for fun, seeing what I could dig up.
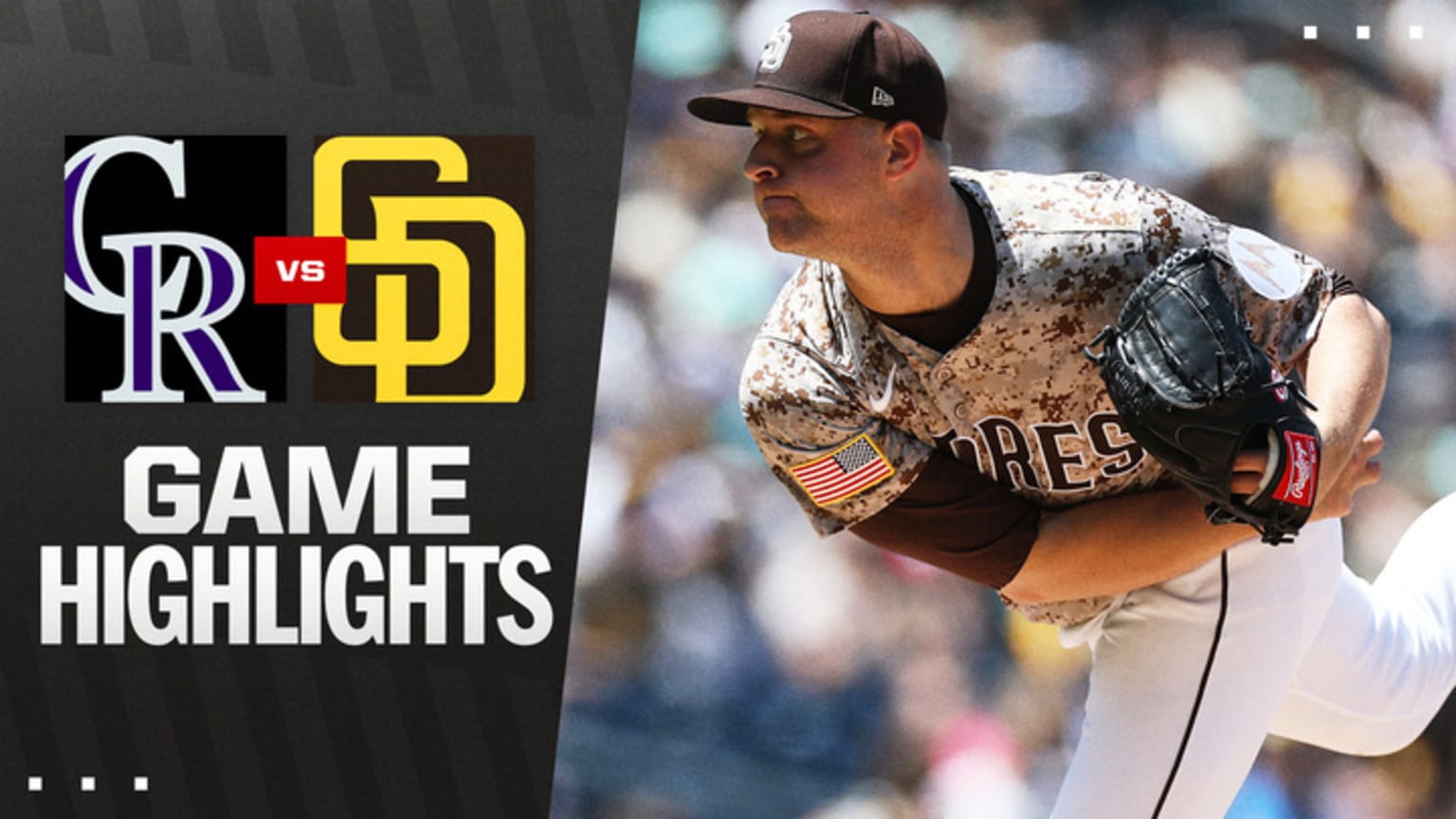
First thing I did was hit up the usual sports stats sites. ESPN, *, the usual suspects. I was looking for a clean table of player stats – batting averages, RBIs, home runs, all that jazz. Sometimes they make it easy, sometimes it’s a pain.
Anyway, I found a decent table on one of the sites, but it was all wonky and formatted weird. Copying and pasting it into a spreadsheet was a disaster. That’s when I was like, “Alright, time to get a little more technical.”
I remembered seeing some Python libraries for web scraping, so I figured I’d give that a shot. I started by installing Beautiful Soup and Requests. Pretty standard stuff, right?
pip install beautifulsoup4 requests
Then, I fired up my trusty Jupyter Notebook and started coding. I used Requests to grab the HTML content of the webpage with the stats table. Then, I fed that HTML to Beautiful Soup to parse it. This is where things got a little tedious.
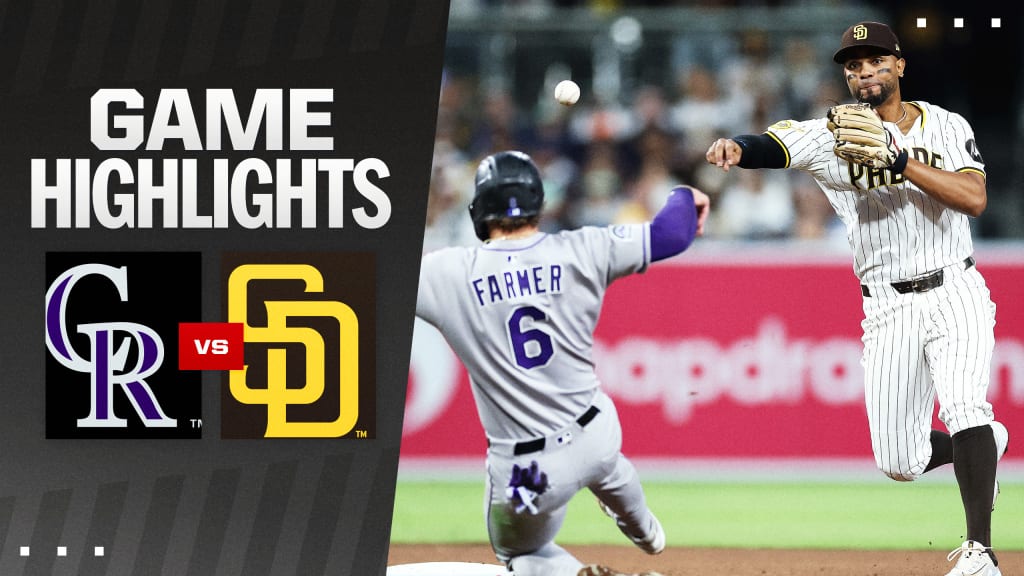
Finding the right HTML tags that contained the player stats was a bit of a hunt. I had to inspect the page source in my browser and figure out which tables and divs held the data I wanted. It’s always a game of trial and error.
After a bunch of digging, I managed to isolate the table with the Rockies and Padres player stats. Then, I looped through the table rows, extracting the player names and their stats for each category. It was kinda messy because there were header rows and other junk I had to skip over.
I ended up storing the data in a Python dictionary, where the keys were the player names, and the values were dictionaries of their stats. Something like this:
{'Charlie Blackmon': {'AVG': '.280', 'RBI': '2', 'HR': '1'}, ...}
Once I had the data in that format, it was pretty easy to dump it into a Pandas DataFrame. That made it way easier to analyze and sort the stats. I could quickly see who had the most RBIs, the highest batting average, etc.
I even tried visualizing the data with Matplotlib. I made a few bar charts showing the top performers in different categories. Nothing fancy, just some quick and dirty plots to get a visual overview.
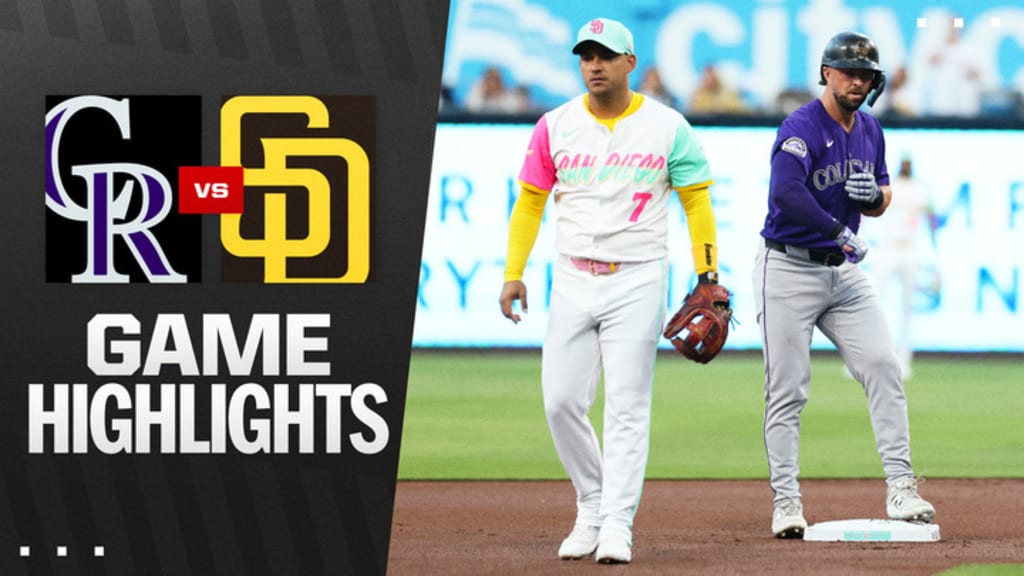
In the end, it wasn’t super polished or anything, but I managed to extract the player stats from that Rockies-Padres game and play around with them a bit. It was a fun little project, and I learned a few things about web scraping and data analysis along the way.
Key Takeaways
- Web scraping can be a bit of a pain, but it’s a useful skill to have.
- Beautiful Soup and Requests are your friends.
- Pandas DataFrames make data analysis much easier.
- Don’t be afraid to get your hands dirty and experiment.
Next time, I think I’ll try to automate the process a bit more. Maybe set up a script to automatically fetch the stats after each game. That would be pretty cool.