Okay, so here’s the deal. You know Urban Meyer, right? The football coach? Well, I got kinda obsessed with his play-calling philosophy. Not to become a football coach, obviously, but to see if I could apply his principles to… coding! Yeah, I know, sounds crazy. But hear me out.
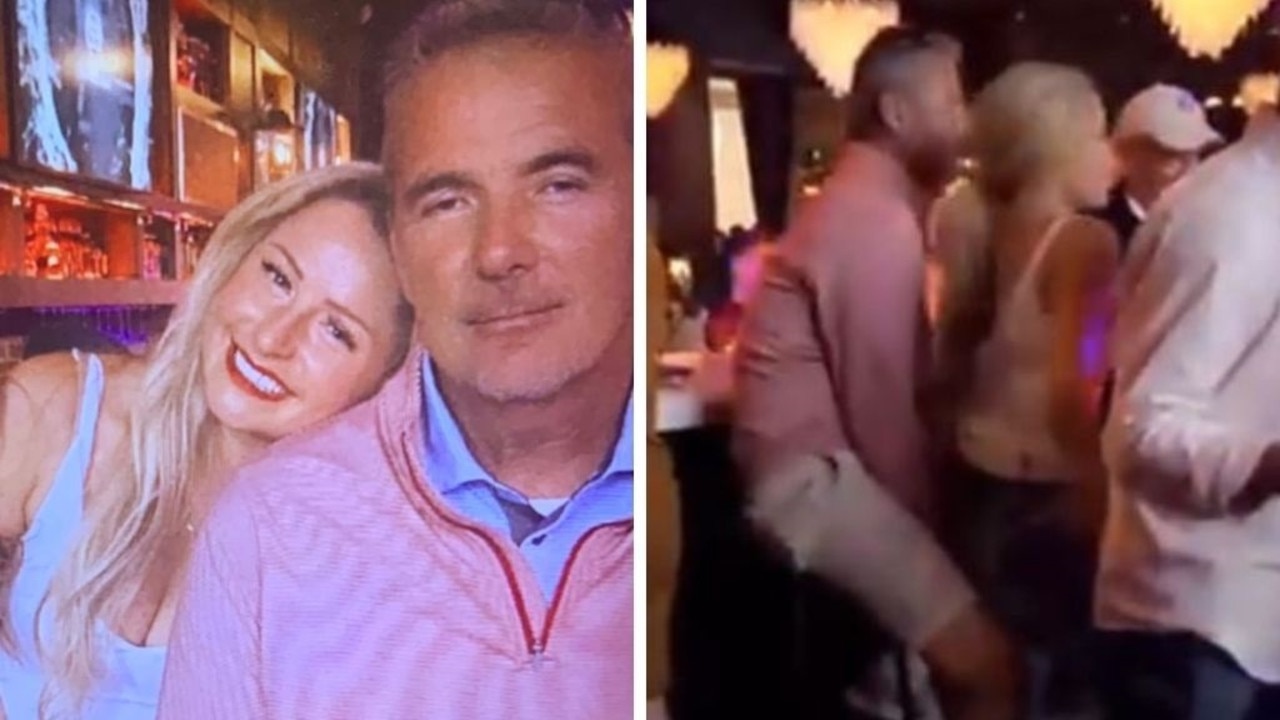
It all started with me reading about his “cheat” plays. The idea is to have a few super-reliable plays that your team can execute perfectly, even under pressure. Plays that the defense knows are coming, but can’t stop. I thought, “What if I had a ‘cheat’ function in my code?” Something that was so well-written, so optimized, and so thoroughly tested that I could drop it into any project and it would just work.
So, first thing’s first, I had to pick a function. After a lot of thinking, I settled on a generic sorting algorithm. Sorting is everywhere, right? And I figured I could really nail it. I started by researching different sorting algorithms – quicksort, mergesort, heapsort, the whole shebang. I spent like a week just reading articles and watching videos.
Next, I coded up a few different versions in Python. I know, Python isn’t exactly known for speed, but it’s great for prototyping. I timed them all, ran them on different datasets, and tried to find their weaknesses. Turns out, quicksort is usually the fastest, but it can fall apart if the data is already partially sorted. Mergesort is slower, but more consistent. Heapsort is… well, it’s there.
Then I started optimizing. I tried different pivot selection strategies for quicksort. I messed around with insertion sort for small sub-arrays (a common trick). I even tried writing a hybrid algorithm that switched between quicksort and mergesort depending on the data. It was a total rabbit hole.
After weeks of tinkering, I had a sorting function that I was pretty happy with. It wasn’t the absolute fastest in every situation, but it was consistently good across a wide range of inputs. I wrote a ton of unit tests, making sure it handled edge cases and weird data. I even ran it through a static analyzer to catch any potential bugs.
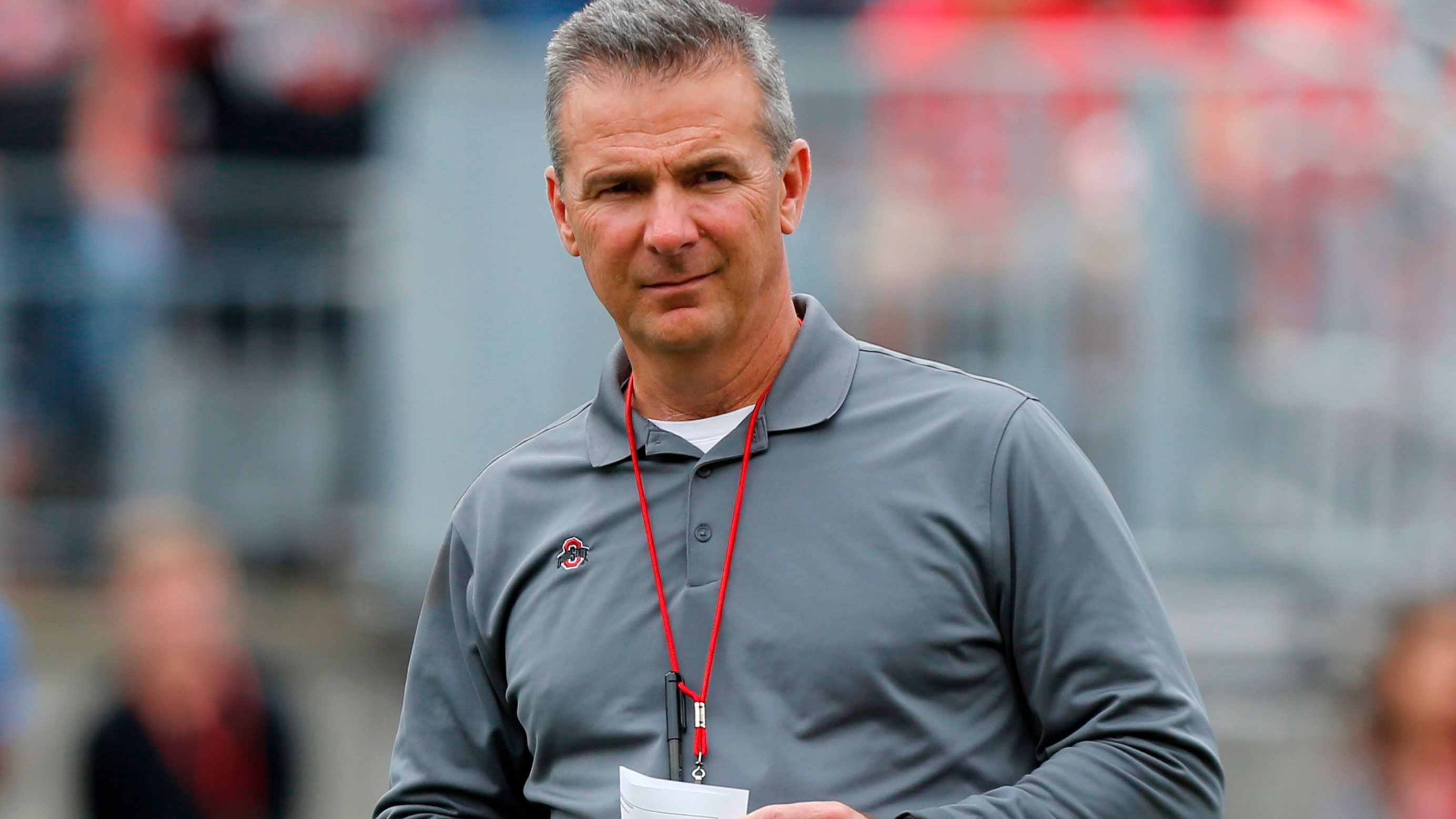
But here’s the key. I then refactored the heck out of it. Made the code super readable and well-commented. Broke it down into smaller, more manageable functions. The goal wasn’t just to make it fast, but to make it easy to understand and maintain. I wanted something that another developer could pick up and use without scratching their head.
Finally, I packaged it up as a small Python module with a clear API. I even wrote some documentation, explaining how to use it and what its limitations were. The whole thing was maybe a few hundred lines of code, but it was my “cheat” play. A piece of code that I knew inside and out, and that I could trust to get the job done.
Has it worked? Yeah, actually. I’ve used it in a few different projects now, and it’s saved me a ton of time. More importantly, it’s given me a little bit of confidence. Knowing that I have this one thing that I can always rely on, it makes me feel better about tackling bigger, more complex problems.
So, maybe Urban Meyer was onto something. Find your “cheat” play, master it, and use it to your advantage.