Alright, let’s talk about how I tackled getting the Phoenix Open leaderboard data. It was a bit of a grind, but hey, that’s how you learn, right?
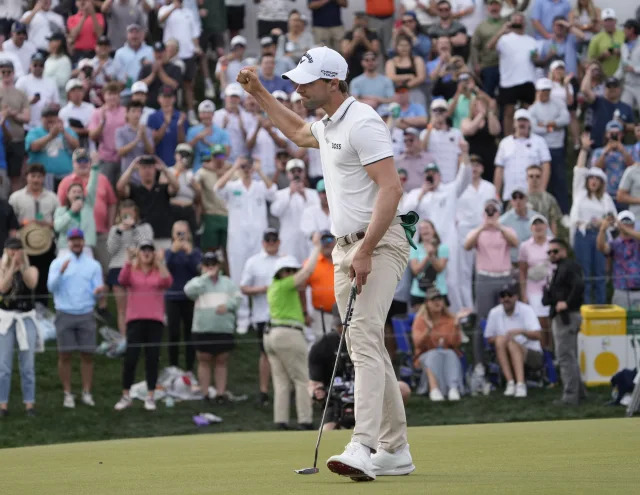
First things first: I needed the data. Obvious, I know, but finding a reliable source was the initial hurdle. I started by just googling “Phoenix Open leaderboard API.” No luck. Most results were just links to sports news sites or official PGA Tour pages. Helpful for viewing the leaderboard, not so much for getting the raw data.
Then I thought, “Okay, maybe I can scrape it.” I checked out the PGA Tour’s official website. The leaderboard was nicely formatted, but it was dynamically loaded. This meant simple HTML scraping wouldn’t cut it. I needed something that could execute JavaScript.
Next up: Puppeteer (or something similar). I fired up * and installed Puppeteer. For those who don’t know, Puppeteer lets you control a headless Chrome browser. Basically, you can programmatically navigate to a website, wait for the page to load, and then extract the data you need. Sweet!
So, I wrote a little script:
- Launch a new browser instance.
- Navigate to the Phoenix Open leaderboard page on the PGA Tour website.
- Wait for a specific element to load (to ensure the JavaScript had finished rendering the leaderboard). This is important!
- Use `*()` to run JavaScript code within the context of the browser page. I used this to extract the player names, positions, scores, etc., from the DOM.
- Close the browser.
The tricky part: Figuring out the right CSS selectors to target the leaderboard elements. The PGA Tour website wasn’t exactly built with scrapers in mind. I had to use Chrome’s developer tools to inspect the HTML and identify the specific classes and IDs that contained the data I wanted.
Data Wrangling: Once I had the raw data, it was a bit of a mess. Some scores were strings, others were numbers. Some players had DNFs, WDs, or other abbreviations in their scores. I wrote some JavaScript functions to clean and transform the data into a more usable format, like an array of objects, where each object represented a player and their stats.
Storage: I needed a place to store this data. For this simple project, I just wrote it to a JSON file. But in a real-world scenario, you’d probably want to store it in a database like MongoDB or PostgreSQL.
Possible alternative: While scraping worked, I kept looking for a proper API. Sometimes these things are hidden. I started digging around in the network requests made by the PGA Tour website (again, using Chrome’s dev tools). Bingo! I found a request that returned the leaderboard data in JSON format. It was undocumented, but it was there! This was way easier than scraping. If you can find an API, even an undocumented one, always prefer that over scraping.
Final thoughts: Getting the Phoenix Open leaderboard data wasn’t rocket science, but it did require a bit of persistence and problem-solving. I started with the obvious (Googling for an API), then moved to scraping when that didn’t work. In the end, I stumbled upon a hidden API, which was the cleanest solution. The key takeaway is to explore all your options and don’t be afraid to get your hands dirty!
Also, remember to be respectful when scraping or using undocumented APIs. Don’t hammer the server with requests, and always abide by the website’s terms of service.
